By: Team T17-2
Since: Aug 2019
Licence: MIT
1. Setting up
Refer to the guide here.
2. Design
This section then entire design structure of TravEzy.
2.1. TravEzy Architecture
TravEzy boasts a plethora of features which includes:
-
Calender
-
Itinerary
-
Financial Tracker
-
Diary
-
Address Book
Due to the numerous features that TravEzy flaunts, the integration of these features requires multiple classes to be implemented.
Here is the Architecture Diagram which explains the high-level design of the App.
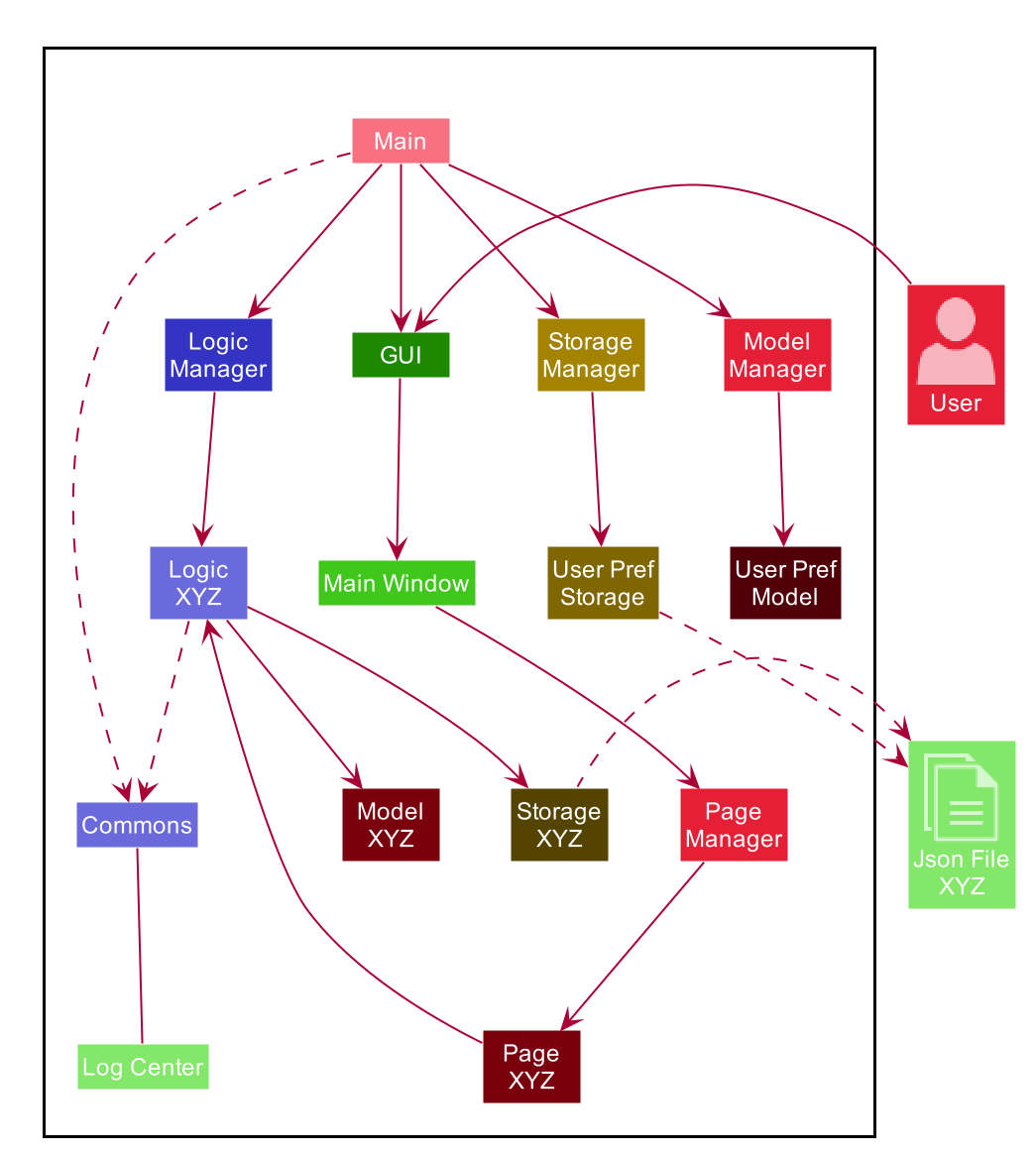
TravEzy is mainly a Common Line Interface (CLI) desktop application. Hence, users will just need to interact with the text UI to trigger responses from Travezy. |
As shown in Figure 1., TravEzy is a desktop application catered only for a single user. Below is a breakdown on how each component work together to make TravEzy possible.
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components.
The following class plays an important role at the architecture level:
-
LogsCenter
: Used by many classes to write log messages to the App’s log file.
Each of the main feature in TravEzy implements their own Page
, Logic
, Model
and Storage
class.
These are represented by the respective XYZ
classes for each component.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
In order to ensure that TravEzy is presented as one integrated app, these individual models are mend by their respective
Manager
.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
2.2. UI component
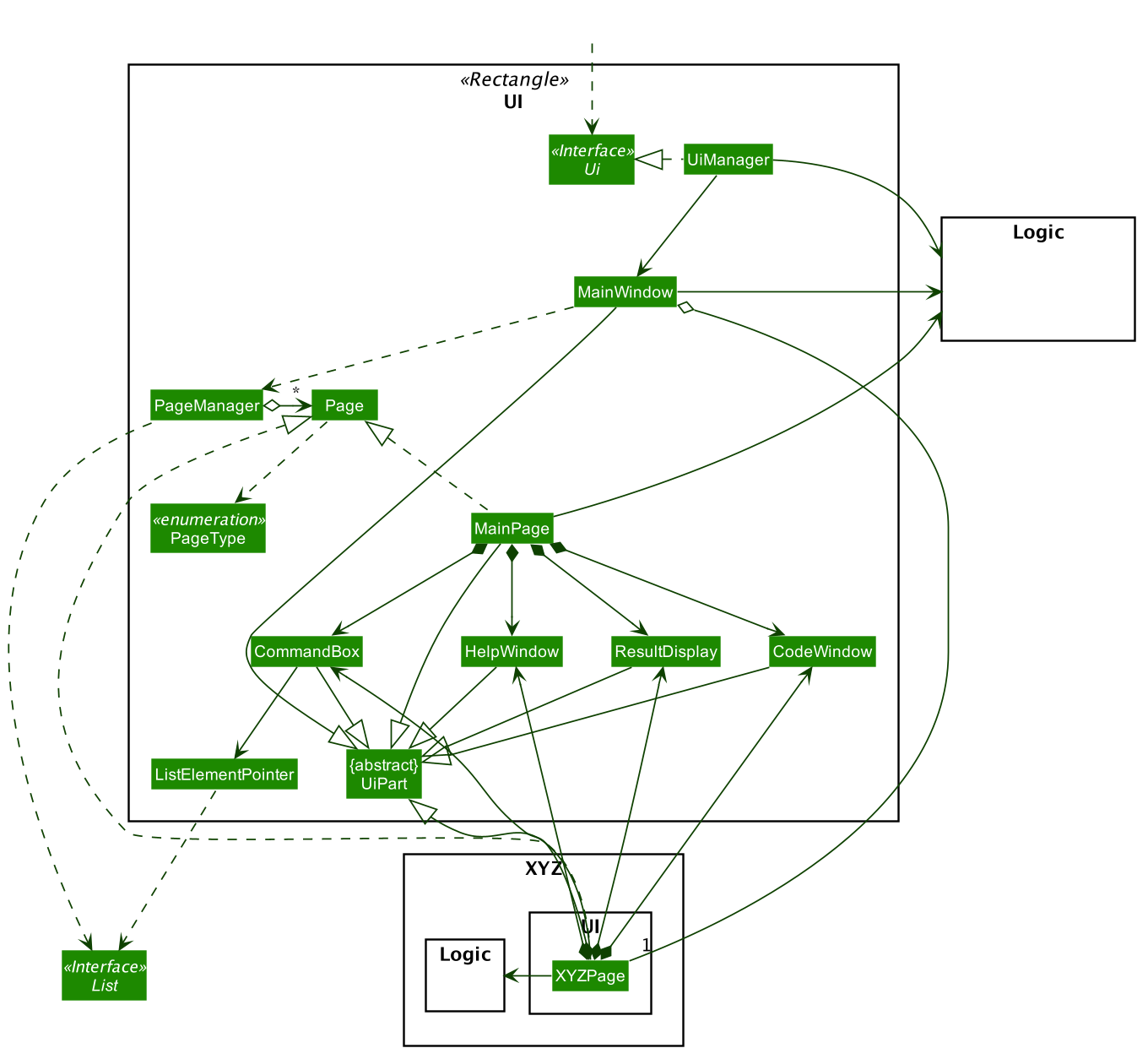
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
In addition, the UI
component,
-
Facilitate the changing of scenes between different user interface for the different features
-
Gets the requested page
-
Changes the scene on the primary stage upon request
-
Executes user commands using the
Logic
component. -
Listens for changes to
Model
data so that the UI can be updated with the modified data.
2.3. Logic component
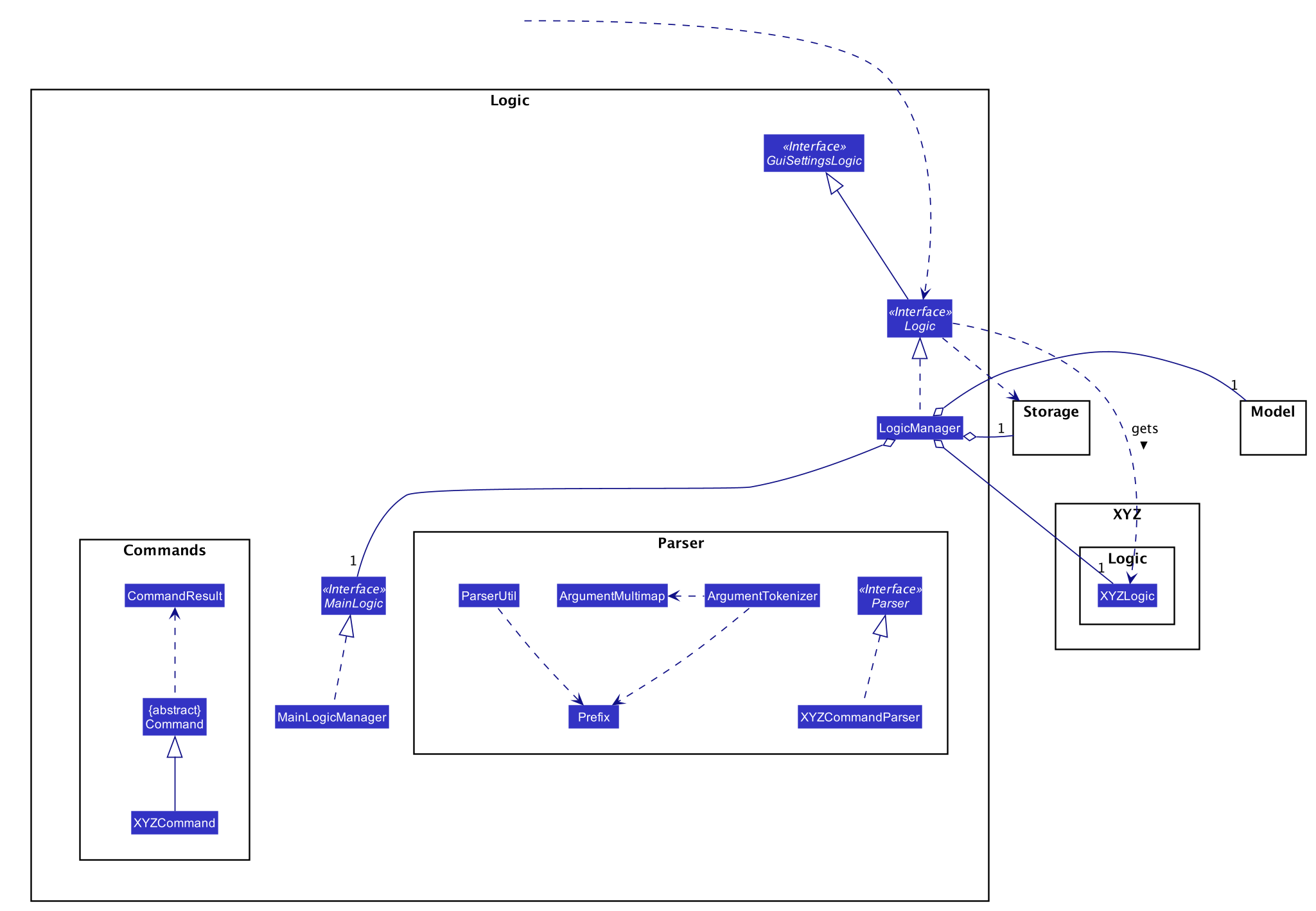
API :
Logic.java
-
Logic
gets theAddressBookLogic
-
AddressBookLogic
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theAddressLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
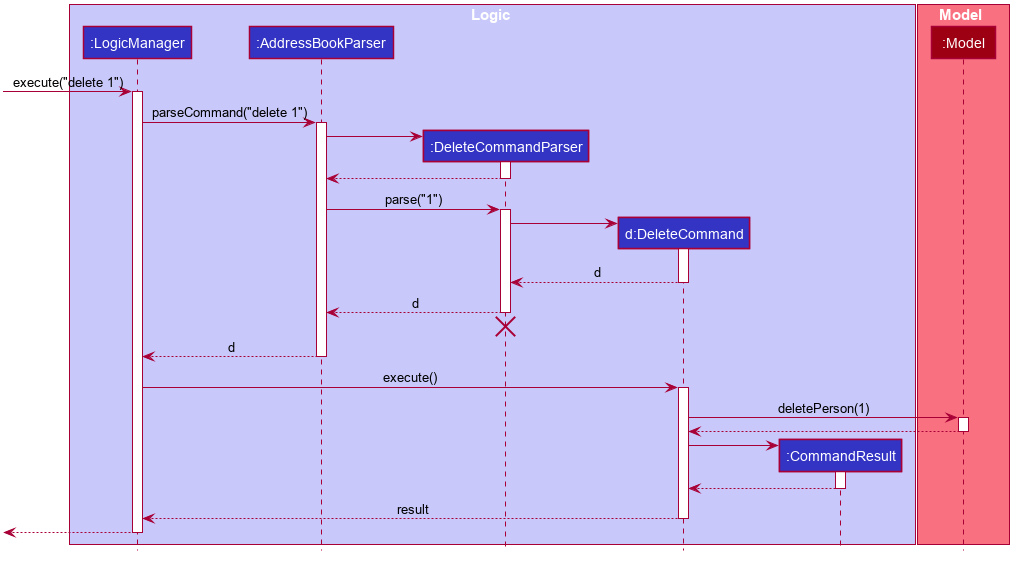
delete 1
Command
The lifeline for DeleteCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
2.4. Model component
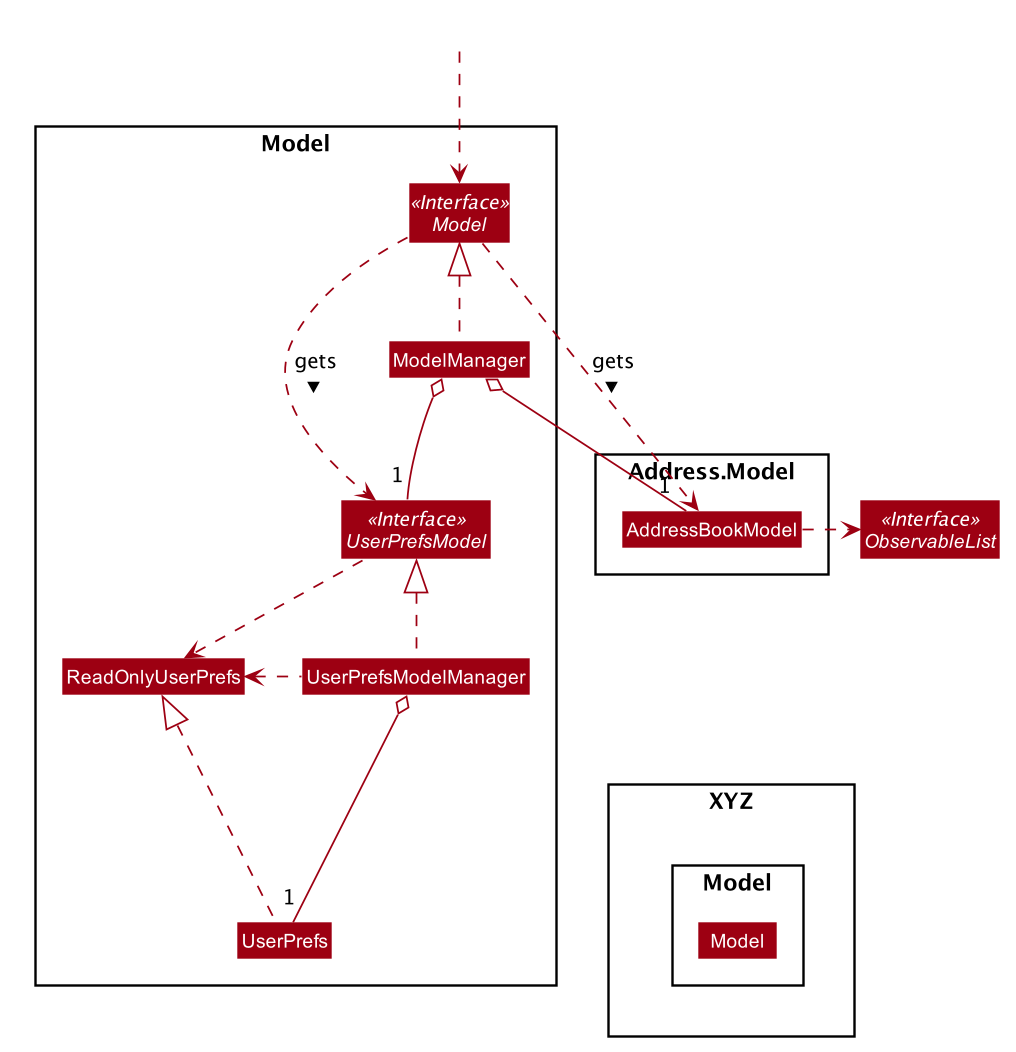
API : Model.java
The Model
,
-
stores the different model used for different packages inside Travezy
-
stores a
UserPrefModel
object used to get and set user preferences -
stores a
AddressBookModel
object used to get data from the address book -
supply a
StatisticsModel
which is only evaluated upon request -
exposes an unmodifiable
ObservableList<Person>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
As a more OOP model, we can store a Tag list in Address Book , which Person can reference. This would allow Address Book to only require one Tag object per unique Tag , instead of each Person needing their own Tag object. An example of how such a model may look like is given below.![]() |
2.5. Storage component
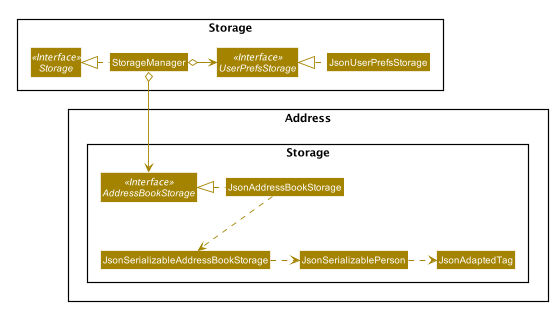
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Address Book data in json format and read it back.
2.6. Common classes
2.6.1. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 2.6.2, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
2.6.2. Configuration
Certain properties of the application can be controlled (e.g user prefs file location, logging level) through the configuration file (default: config.json
).
3. Features
TravEzy has several features which are listed below. Each of these features have their own design and implementation logic. === Calendar
The calendar feature in TravEzy allows users to easily plan when to travel. In this section, we will discuss how the calendar is designed and how the add an event feature is implemented.
3.1. Calendar Model
This section discusses some design details of the calendar model.
The following diagram illustrates the class diagram of the most important part of the calendar model. Specifically, it focuses on the Event
component.
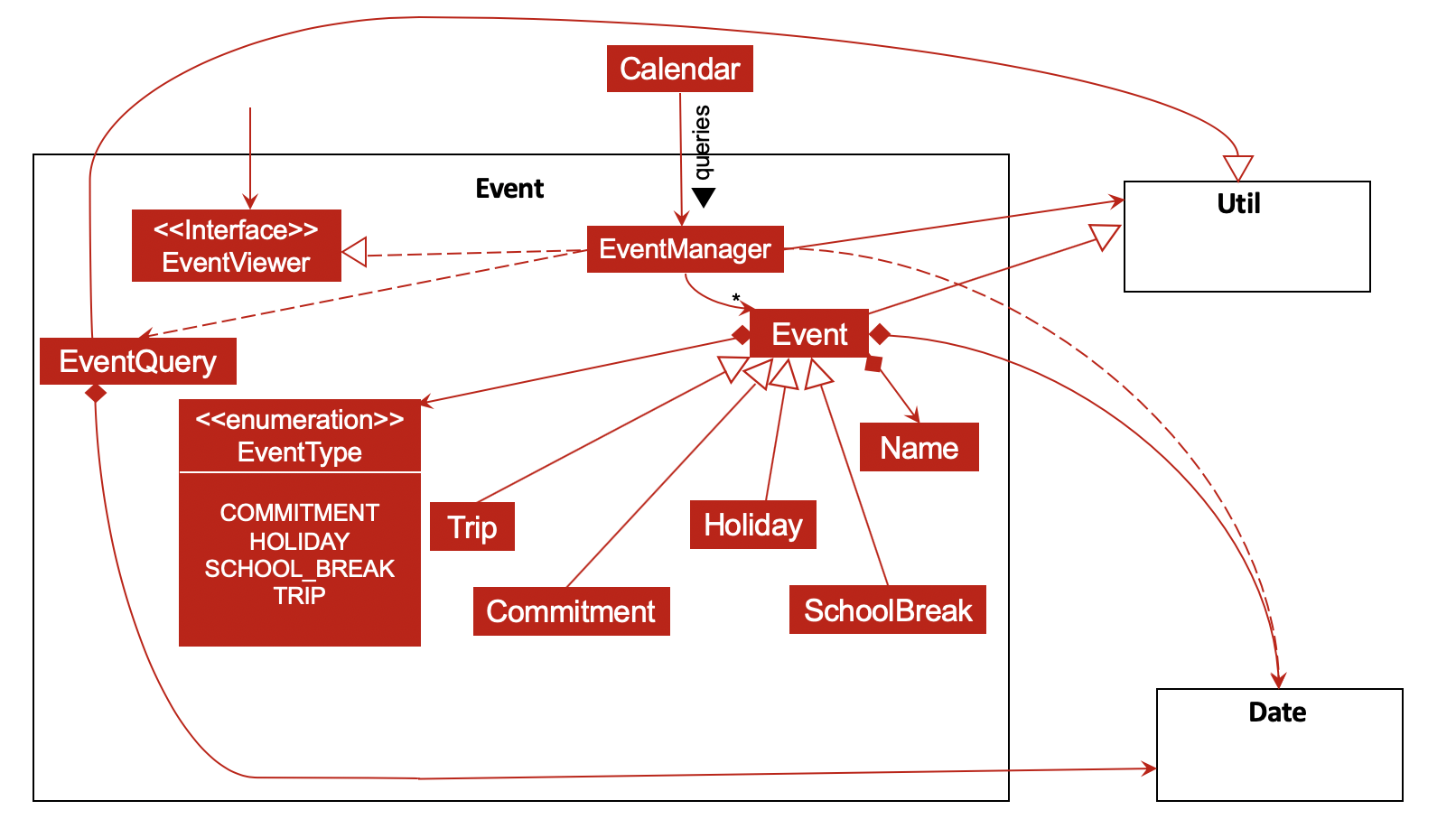
As shown above, the Calendar
instance interacts with the EventManager
by querying it. With the help of EventManger
, the Calendar
instance does not need to deal with "lower-level" concerns (for instance, how Event
s are managed internally).
Every EventManager
can access Event
s that have been added. These Event
s can be Commitment
, Trip
, SchoolBreak
or Holiday
instances. If other instances need a read-only access to EventManager
, they can obtain it using the observer EventViewer
which is implemented by EventManager
.
In addition, every EventManager
depends on EventQuery
to perform queries.
More information about this package is provided below.
What is an Event
?
-
represents the event specified by the user: it is made up of the relevant
Name
,EventType
andDate
(which is found in theDate
package) instances
What is an EventQuery
?
-
similar to an
Event
but is only made up ofDate
-
is used to query the
IntervalSearchTree
(from theUtil
package) to obtain events that are relevant to the user’s request more efficiently.
How do classes in Util
interact with those from Event
?
-
Util
contains important classes and interfaces likeDateUtil
,IntervalSearchTree
andInterval
-
DateUtil
helpsEventManager
to deal with manipulation ofDate
-related operations -
IntervalSearchTree
makes certain user-requested operations more efficient computationally. However, to facilitate the use ofIntervalSearchTree
,Event
andEventType
need to inherit fromInterval
.
To find out how Calendar
, EventManager
and IntervalSearchTree
described above interact, consider the following sequence diagram. It describes how these classes work together when the user tries to add a holiday, which is represented by event
(a Holiday
instance) in the diagram below.
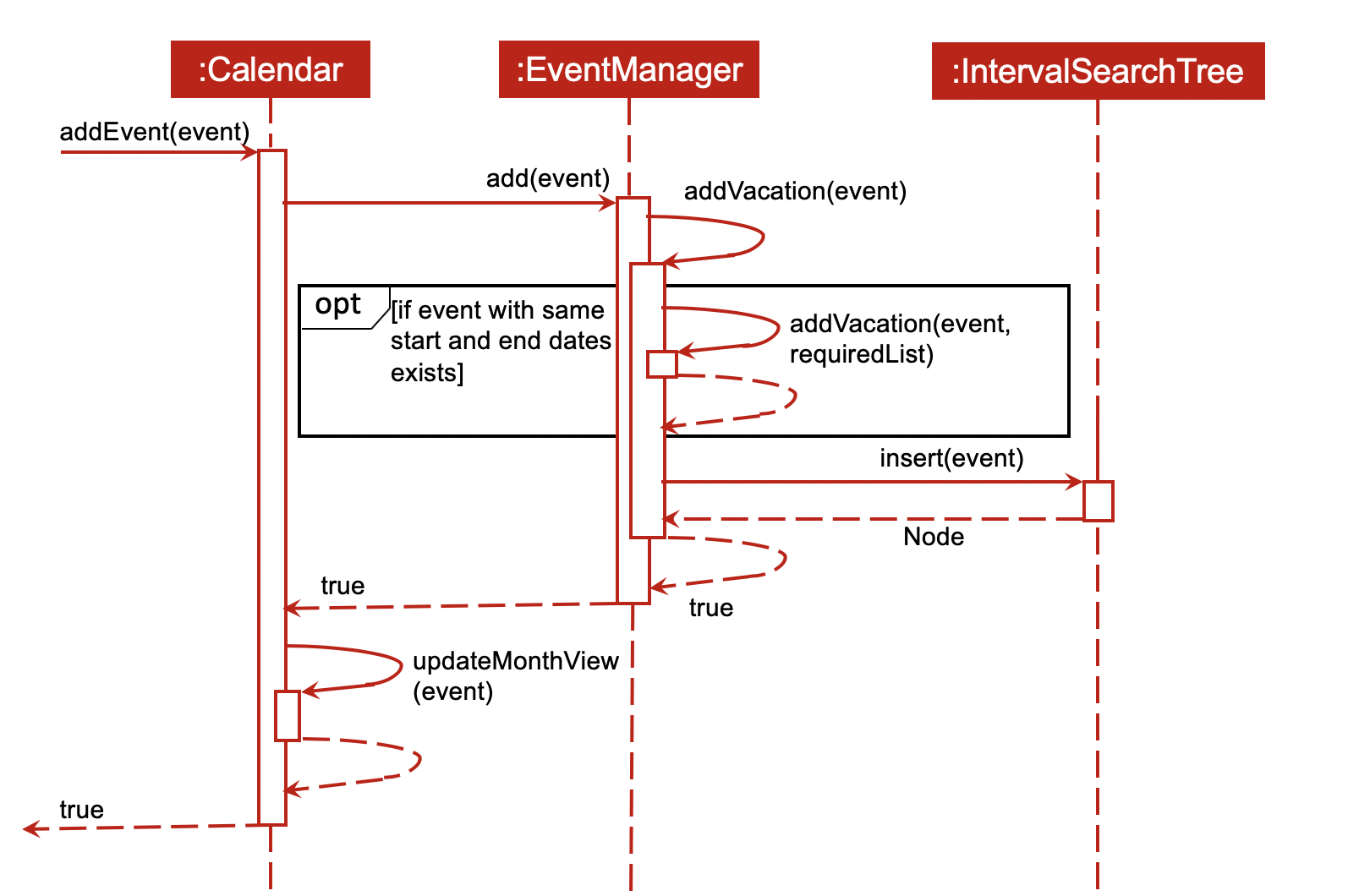
To simplify the sequence diagram, the process of validating that event is in fact a Holiday instance has been left out.
|
3.2. Add An Event Feature
This section provides some details about how the add an event feature is implemented.
The following diagram illustrates the course of action(s) that is(are) possibly taken when a user tries to add a new trip to his/her calendar.
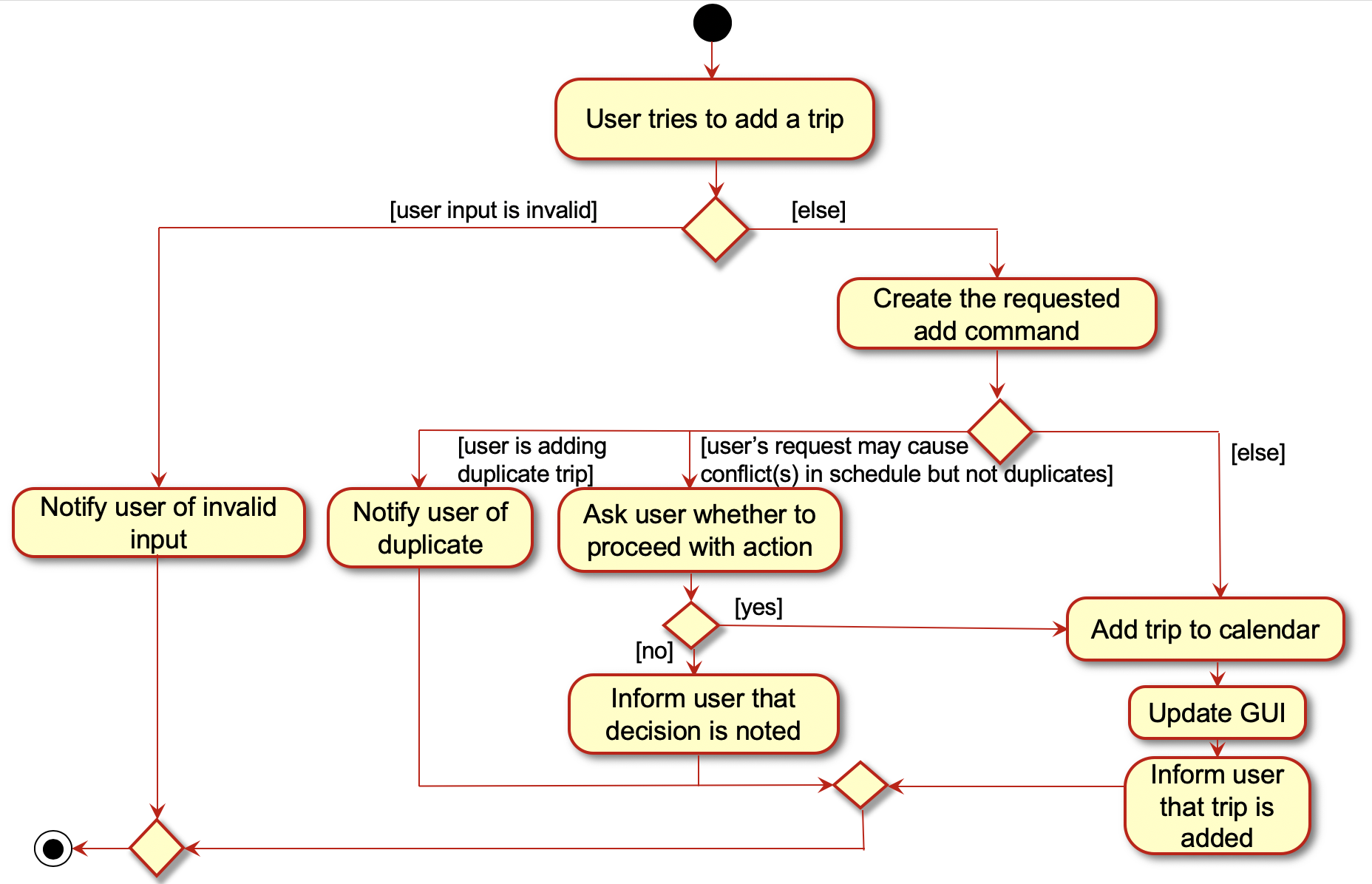
From the diagram, it can be seen that there are three major reasons that can interrupt the user request:
-
the user entered an invalid input (for instance, by typing an invalid date)
-
the user tried to add a duplicate event
-
the user tried to add an event that may result in conflicts in his/her schedule
3.3. Design Considerations
This section details a few design considerations I made before deciding how I would like to implement the functionalities of this calendar.
Aspect | Alternative 1 | Alternative 2 |
---|---|---|
Data structure to support |
The calendar simply stores all events in a list.
|
The calendar stores the events in an interval search tree.
|
Required date format for user input |
The usual date format like dd/mm/yyyy (for example, 12/05/2019 to specify 12 May 2019).
|
Allow users to specify day, month and year in whichever order they want.
|
3.4. Itinerary
The itinerary feature in TravEzy allows users to organize their events and view these events in one convenient Event List.
Current, the itinerary feature supports the basic commands of add, delete, edit and marking your events as done. In addition, it also includes other more advanced commands such as search and sort to better organize your events. With these implementations, TravEzy aims to be at the frontier of convenience.
The itinerary feature implements the aforementioned feature based on the use cases below:
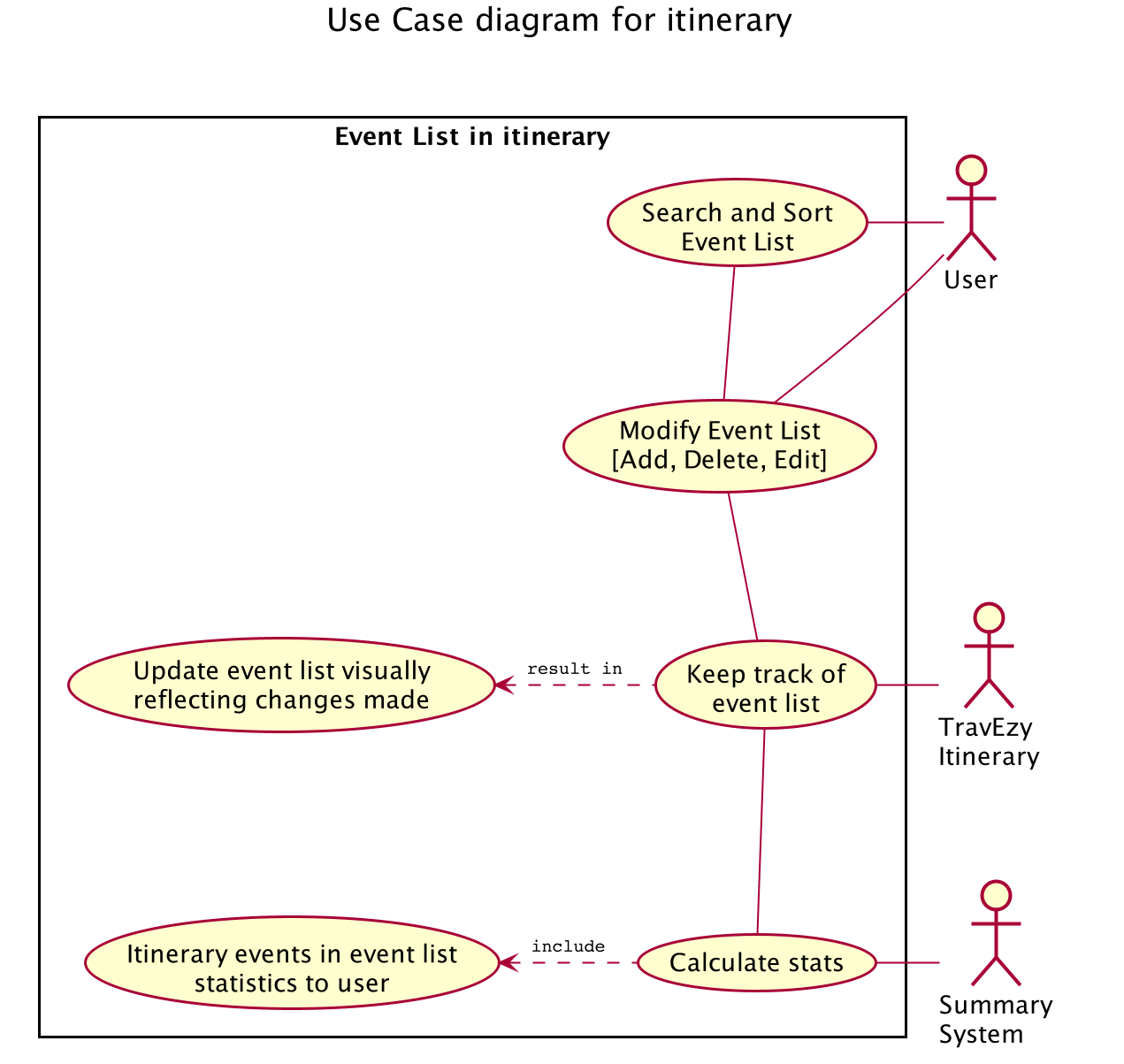
API :
ItineraryLogic.java
Due to the numerous features supported by the itinerary, it requires a complex structure to ensure that each input by the user are cautiously parsed before giving the appropriate command result.
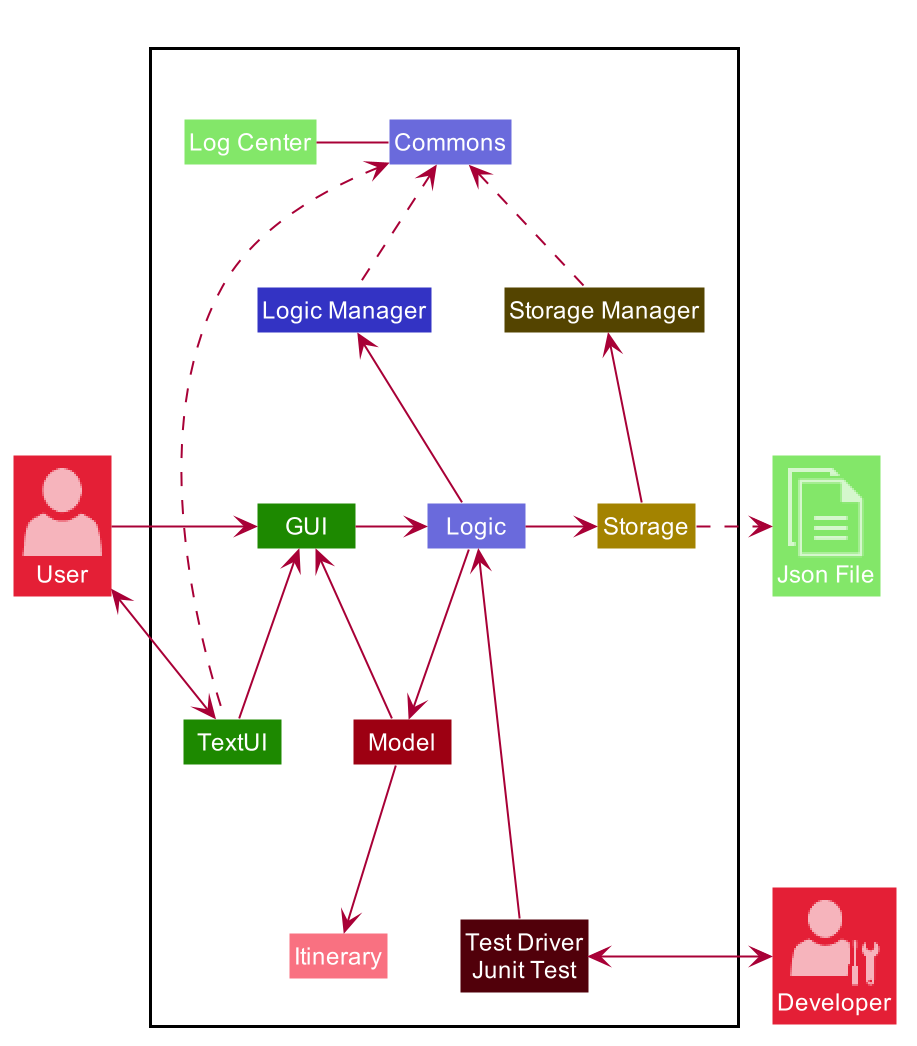
The Architecture Diagram given above explains the high-level design of the itinerary feature. Inputs given by the user are channeled from the text UI and parsed in the logic package before different commands are formed which generates the model and updates the itinerary object which contains the event list.
The text UI
, logic manager
and storage manager
all stem from the common package of the main TravEzy application.
However, in the parser
package of itinerary, it contains various parser objects for the different command.
This is to ensure that each command in the itinerary have only one parser validating the command.
3.4.1. Model Component
The implementation of the model class in TravEzy is to be a generic. Hence, the model object being instantiated
could be any of the following 5 features, Calendar
, Itinerary
, Financial Tracker
, Travel Diary
and Achievements
. Below is the model class diagram for the itinerary feature:
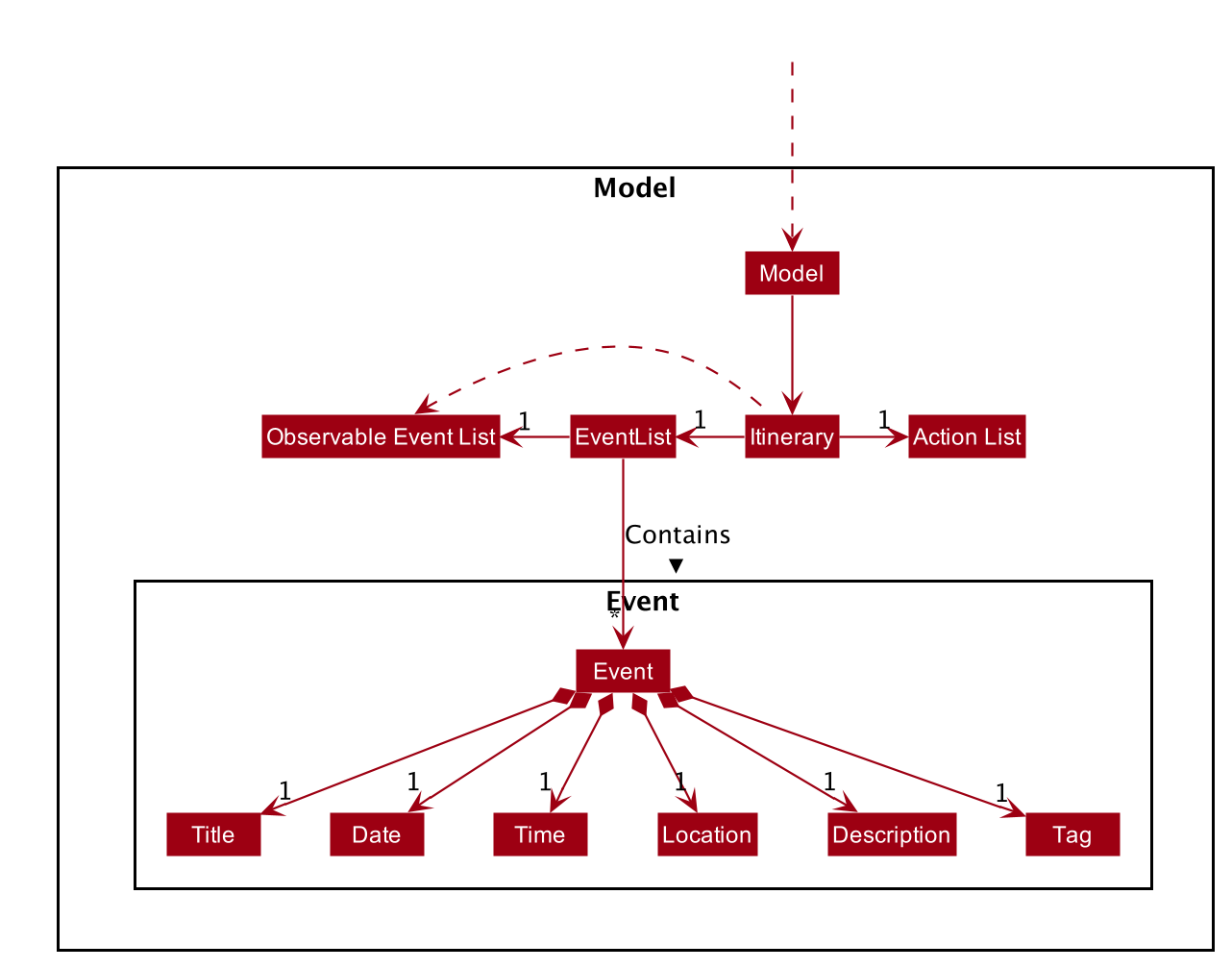
The Model, is the crux of the itinerary feature which serves several functions this include:
-
stores the Itinerary data which includes the event list which keeps track of all the events that are included by the user and stores it into the storage in a JSON file.
-
exposes an unmodifiable ObservableList<Event> that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change.
-
does not depend on any of the other three components, UI, logic and storage which are common through all the features throughout TravEzy.
A plausible extension to the itinerary model is to have the location field dictated by a dropdown bar, similar to the Priority Dropdown Bar. |
Alternative implementation to the proposed extension:
-
Use javaFX drop down menu to select the location of the event:
PROS: Can use location as a search condition and easier for storage / maintenance purposes.
Standardize the UI layout with TravEzy Financial Tracker which also has a Dropdown bar for location.CONS: Limiting the users to only a certain set of locations.
-
Allow user to type in location during the add command template:
add title/[title] date/[date] time/[time] l/[location] d/[description]
PROS: Allows the user to put in any location they want to allow for more variety. Search function is still applicable for location but users would need to know the specific location they used for that particular event.
CONS: Harder to maintain the individual locations in the storage since there could be a lot of locations that are being defined by the user.
3.4.2. Itinerary search command
The search command for specified events in the event list is facilitated by the Itinerary
class which contains
an event list and keep track of the events that the user has inputted into TravEzy. There are several search conditions
available for the users to search from based on the different class attributes that form the Event
class:
-
search title/[title]
-
search date/[date]
-
search time/[time]
-
search l/[location]
-
search d/[description]
Given below is the sequence diagram of how the Itinerary feature interacts with TravEzy when the search command is being called.
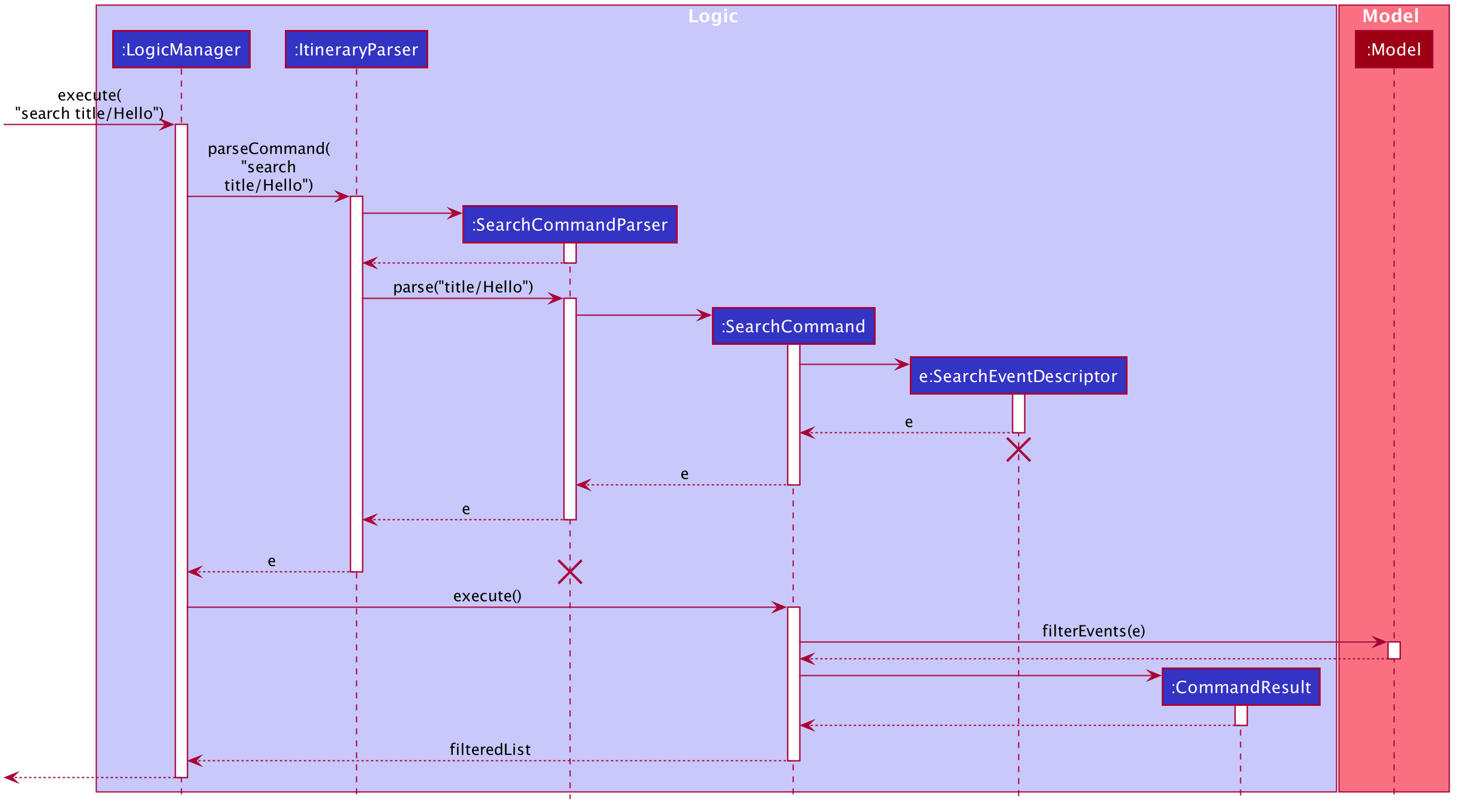
The lifeline for SearchCommandParser and SearchEventDescriptor should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram. |
The search command is implement as follows, upon giving the command by the user in the text UI, the command
will be channeled to the Logic
class where it identifies it as a itinerary command and passes it to the ItineraryParser
class.
The Itinerary Parser
passes through the command into a switch case block and identifies this as a SearchCommand
.
This will create a new SearchCommandParser
which will then accept the arguments from the user’s input and parse the
arguments of the command.
Once the arguments are parsed and considered as valid, the SearchCommandParser
will generate a new SearchCommand
.
The SearchCommand
will in turn create a "pseudo-event" known as the SearchEventDescriptor
which is an event
which only contains attributes with the search condition while the rest of it’s attribute will be placed as null.
This SearchEventDescriptor
will in turn be returned and used in the Predicate
field as the event in comparison.
The filterEvents(e)
method will be called with e
being the SearchEventDescriptor
that is being generated. Events
that are currently in the event list will be filtered accordingly based on whether it matches the attributes in the
SearchEventDescriptor
. Finally, the filteredList
will be generated and returned.
The aforementioned steps could be easily summarized using an activity diagram when the user executes a search command in the itinerary:
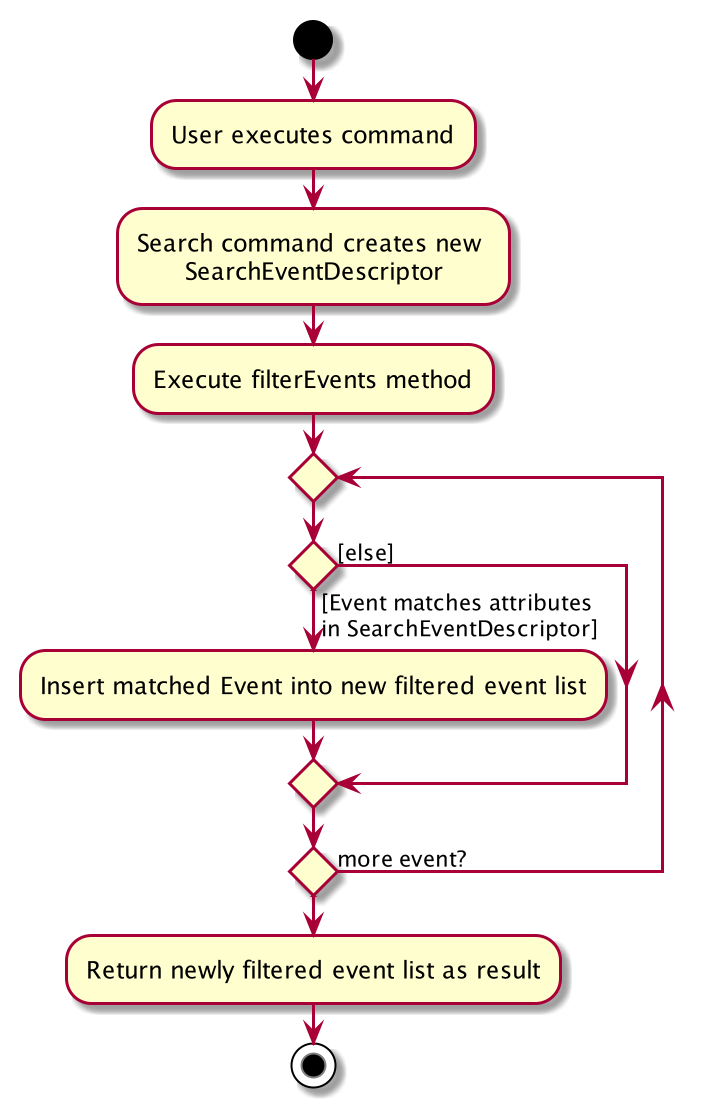
The following search command is implemented in the series of steps as described in the activity diagram as shown above is to ensure that validation of the arguments given in the user command first before the SearchCommand is generated.
Once the arguments from the user input has been verified, the "pseudo-event" created can help to proof-read whether
users have given any conditions for the search. It will return false if all the attributes in the SearchEventDescriptor
.
Running through all the events in the event list will ensure that all the events are being considered in the filtering process.
This sequential approach in implementing the SearchCommand
will ensure that all the current events are being looked through
based on the specific search condition and only the filtered list will be given as a result.
3.5. Financial Tracker
The Financial Tracker feature in TravEzy allows users to keep track of their financial expenses with appropriate categorized expenditure by different countries.
Architecture:
The Architecture Diagram given below explains the high-level design of the Financial Tracker feature.
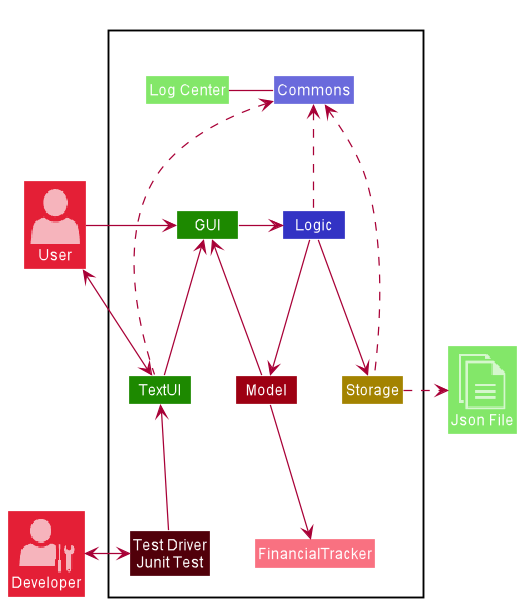
Inputs given by the user are channeled from the textUI
and handled by Logic
before different commands are formed which execute on
the Model
. The Model
will then update the FinancialTracker
object which contains the expense list.
The textUI
and Log Centre
stem from the common package of the main TravEzy application while Logic
, Model,
and Storage
all have similar architecture design with the other features (Calendar, Itinerary, etc.).
Financial Tracker Class Diagram:
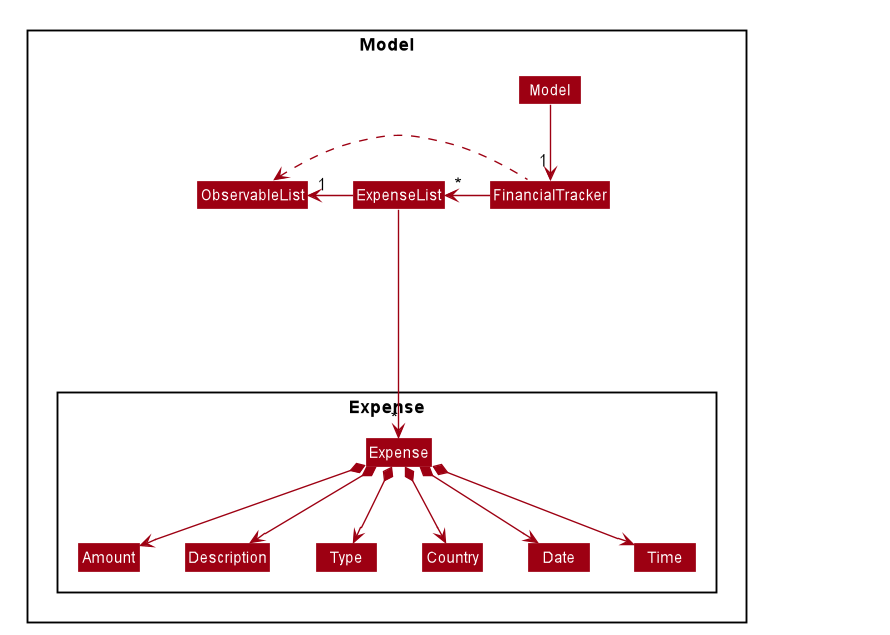
The Model
stores the FinancialTracker
data which includes several ExpenseList
each associated with a country that keeps track of all the expenses.
Financial Tracker
exposes an unmodifiable ObservableMap<String, ExpenseList>
that can be observed. The UI is bound to the Model
whereby the Model
returns an expense list associated with the current Country
of the Financial Tracker
and updates when the querying Country
changed.
Each time a new Country is selected from the Countries dropdown box, the respective expense list will be returned for that specific country, this implementation is to ease categorising and sorting.
|
Sequence Diagram example:
The sequence diagram below shows the sequence of events that will take place when a user calls Summary
command.
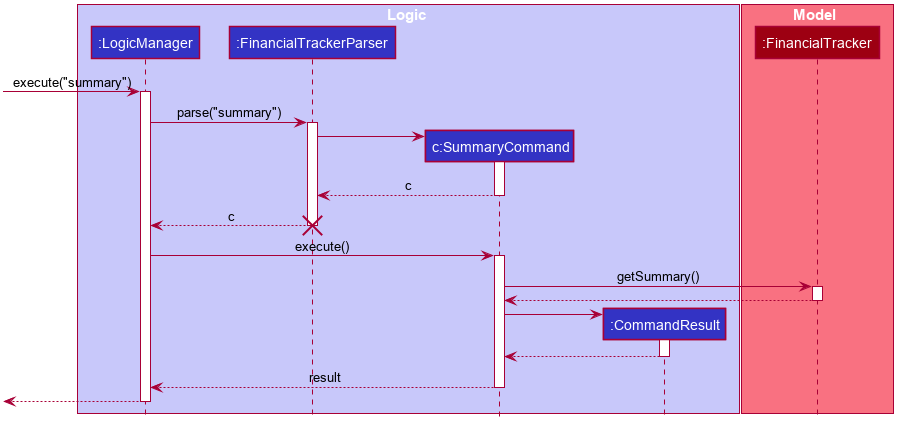
The sequence of events are very likely to those of the other commands in Financial Tracker feature.
Activity Diagram example:
The activity diagram below shows how edits
command works.
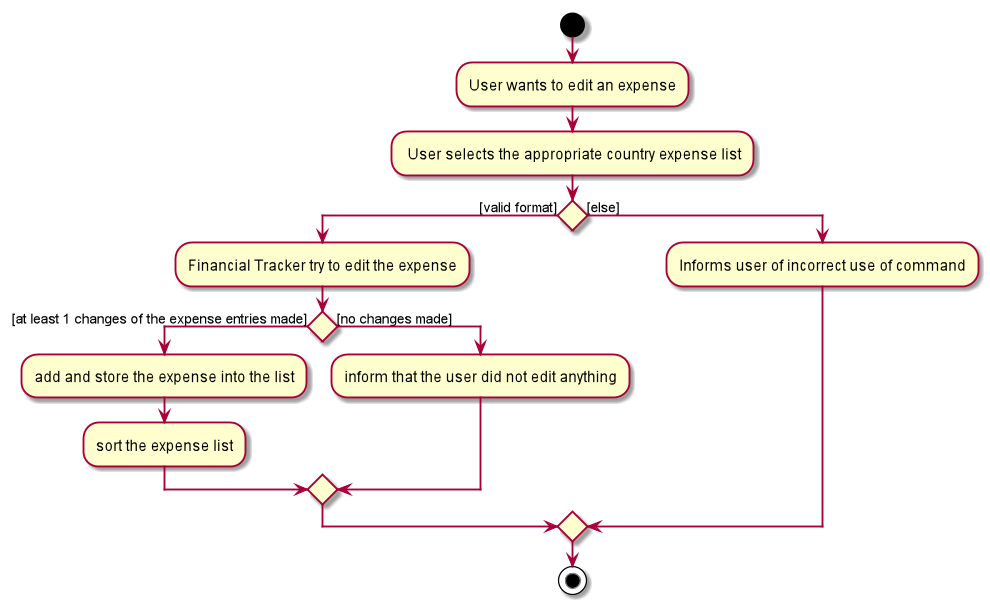
3.6. Diary
3.6.1. Architecture of Diary
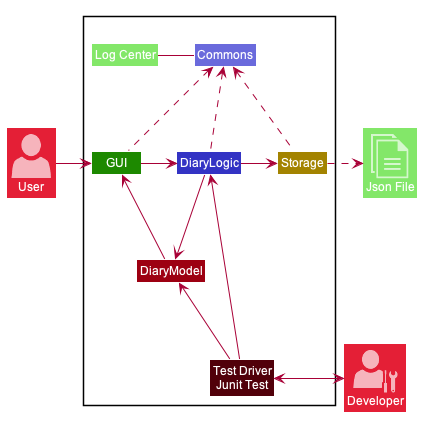
The Architecture Diagram above explains the high -level design of the Diary within TravEzy. Below, there is a brief explanation of each component.
The actual Diary is represented as a DiaryModel
in the diagram. This DiaryModel
has the basic functionalities of a real Diary.
It can store multiple entries which can then be manipulated by the user. The exact functionalities are described in more detail in the Model section below.
GUI
represents the Graphical User Interface through which users input their commands.
DiaryLogic
is the main executor of Diary. It is composed of the Logic
, DiaryParser
and DiaryCommand
. After receiving input from the GUI
, logic triggers
the DiaryParser
to Parse
the input and output the matching Command
DiaryModel
. As mentioned above, this encapsulates a Diary and hence, the Command
from DiaryCommand
is implemented on this DiaryModel
Storage
. After the Command
has been executed, logic then stores the updated DiaryModel`in `Storage
. Data is written to and read from the hard drive using Storage
.
Commons
. DiaryParser
and GUI
are derived from classes in Commons
. Diary Feature also utilises the Log Center
JUnit Test
contains the testing classes for the Diary.
3.6.2. Structure of Diary Model
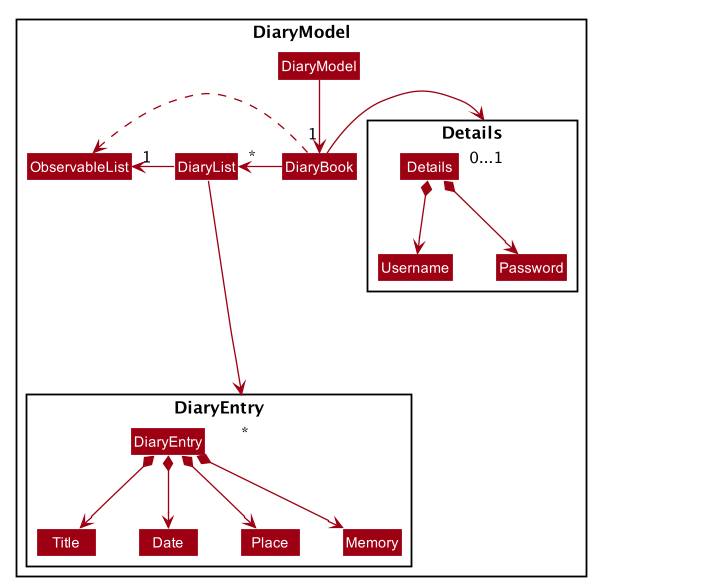
API :
DiaryBookLogic.java
The above diagram is a Class Diagram of the DiaryModel
DiaryModel
Encapsulates the Diary and prevents users from tampering with the underlying structure of the Diary.
It contains the DiaryBook
, which is the actual implementation of Diary.
DiaryBook
is the actual implementation of the Diary.
It contains:
-
DiaryList
, which is a collection of all theDiaryEntries
in a list form -
Observable List
, which exposes an unmodifiableObservableList<DiaryEntry>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates upon any changes. -
Details
, which is a component of the Diary to keepDiaryEntries
private using username and password protection
This DiaryBook
has the relevant methods to allow users to add, delete, find general and specific DiaryEntries
, private and unprivate their DiaryEntries
and also password protect their DiaryEntries
.
DiaryEntries
: This is the base component of the Diary, and encapsulates a single entry into a diary.
It contains:
-
Title
, which refers to the title of theDiaryEntry
-
Date
, which refers to the date and time of theDiaryEntry
-
Place
, which refers to the place of theDiaryEntry
-
Memory
, which refers to the memory of theDiaryEntry
3.6.3. Sequence of Find Command
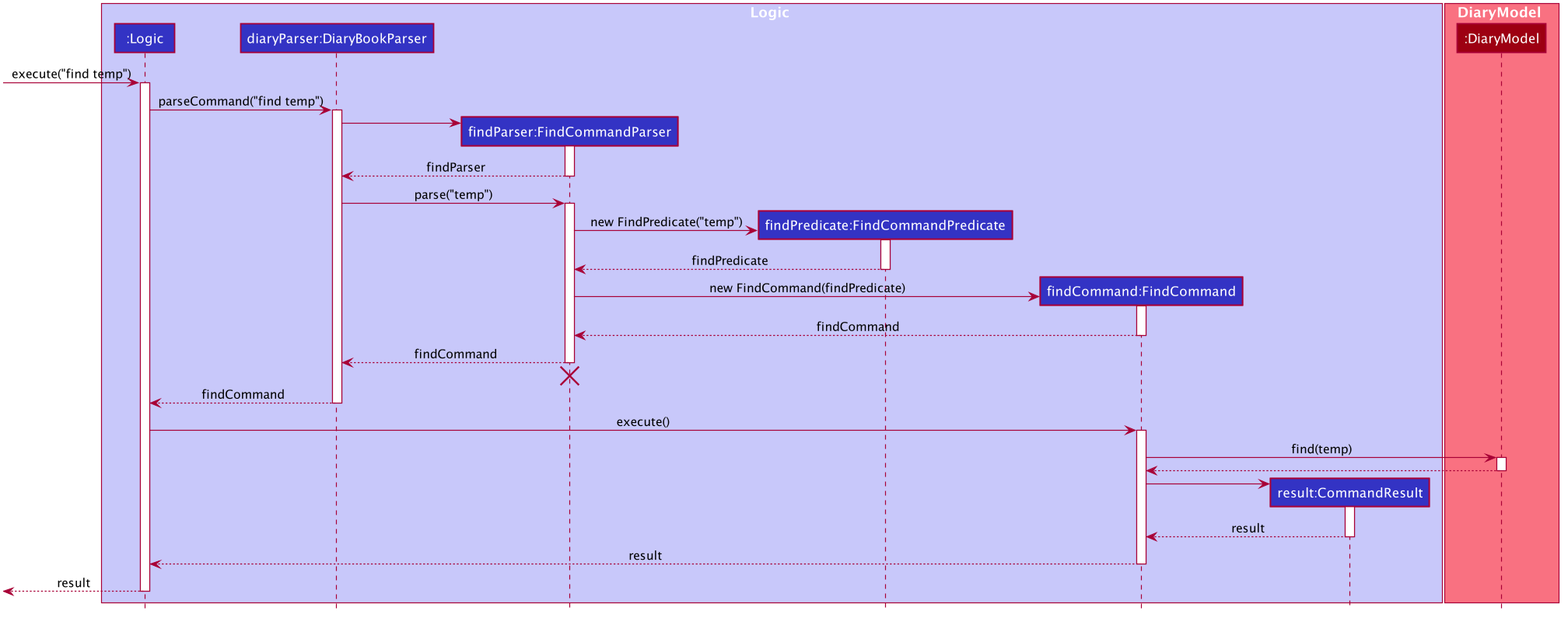
Above, we have the sequence diagram for the FindCommand
in the Diary. In this case, we have taken the scenario of finding any DiaryEntries
which contain the word "temp".
Process:
-
Upon receiving the instruction from
Logic
to parse the inputfind temp
, theDiaryBookParser
begins parsing the input to match it (based on the Command Word) to the appropriate Parser -
In this case, given that the the Command Word is
find
the appropriate Parser is theFindCommandParser
, which is created and then begins toparse("temp")
-
This in turn creates a new
FindPredicate
, based on the inputtemp
. ThisFindPredicate
will be used to filter through theDiaryModel
to get the matchingDiaryEntries
-
With the
FindPredicate
, a newFindCommand
is created which is returned to logic and executed -
This execution leads to the
find(temp)
command being executed on theDiaryModel
, which returns the updatedDiaryModel
with the matchingDiaryEntries
-
This model is then returned to the user in the form of the
Command Result
, and is visible on the GUI in the form of a list of the matchingDiaryEntries
.
The lifeline for FindCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram. |
3.6.4. Logic of Unprivate and Unlock Commands
Given the similar function of the 2 commands, unprivate
and unlock
, there is a more detailed explanation of the differences between the commands below.
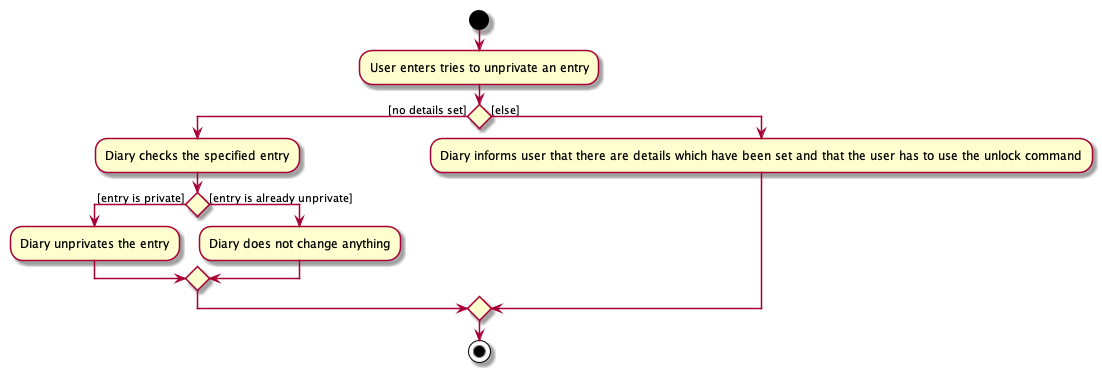
Above, is the logical flow for the Unprivate Command. In brief, it allows a user to unprivate any private entry IF there are no Details
set. As a result, the command can be input like this:
unprivate 1
1 is the index of the Diary Entry which is to be unprivated
|
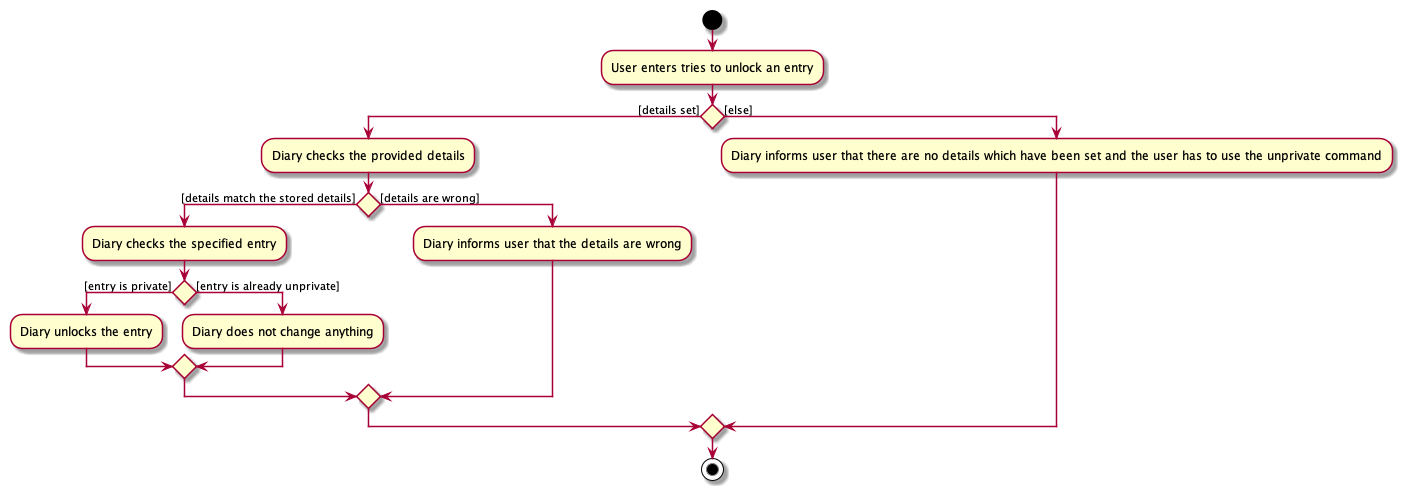
Above, is the logical flow for the Unlock Command. In brief, it allows a user to unlock any private entry IF there are Details
and the Details
input by the user match the original Details
set by the user.As a result, the command can be input like this:
unlock 1 user/username password/password
1 is the index of the Diary Entry which is to be unlocked and user/username and password/password are the relevant Details.
|
In summary, the unlock
command is only to be used when the user has already set Details
. Otherwise, the user has to use the unprivate
command to see private DiaryEntries
3.7. Address Book
3.7.1. Logic Component
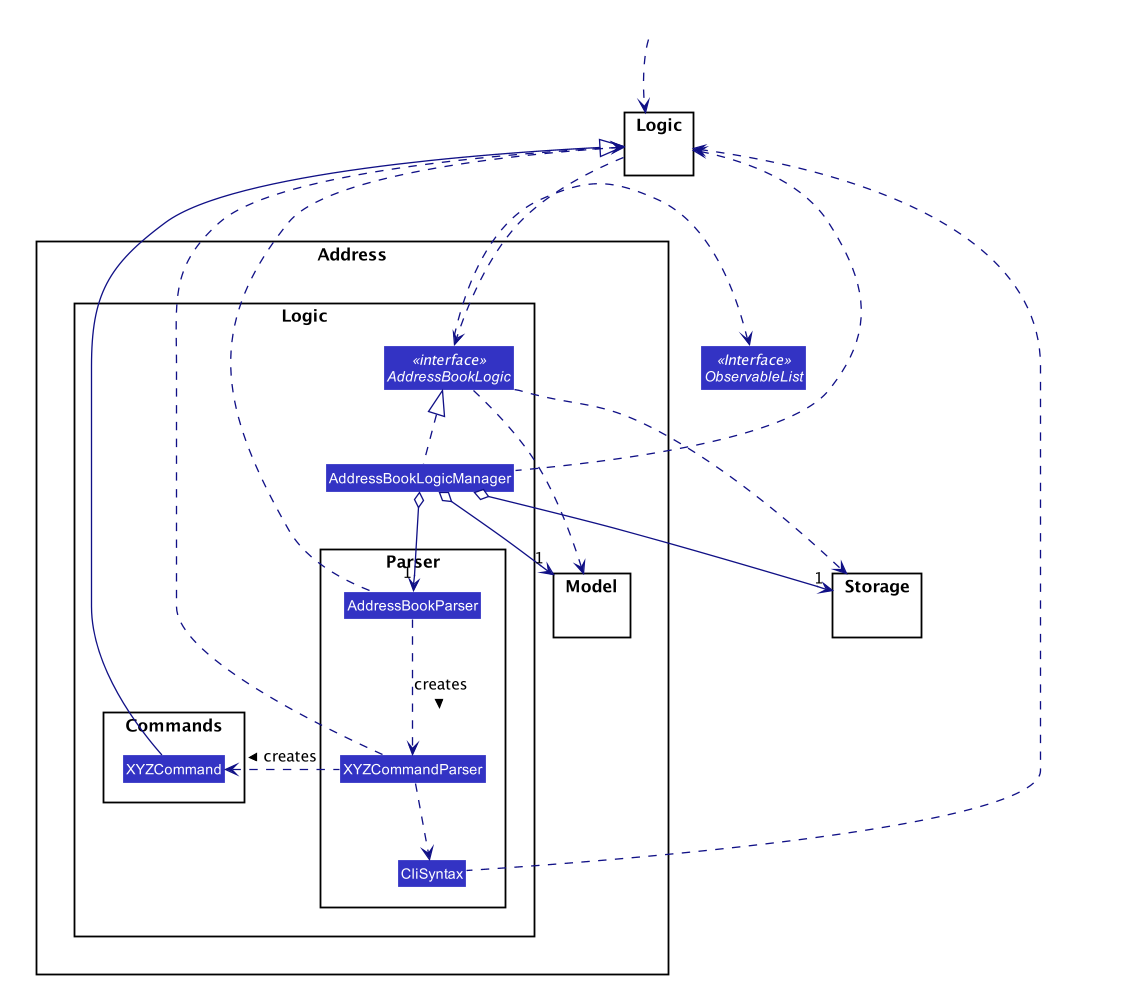
API :
AddressBookLogic.java
-
AddressBookLogicManager
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theAddressBookLogicManager
. -
The command execution can affect the
AddressBookModel
(e.g. adding a person). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theAddressBookPage
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
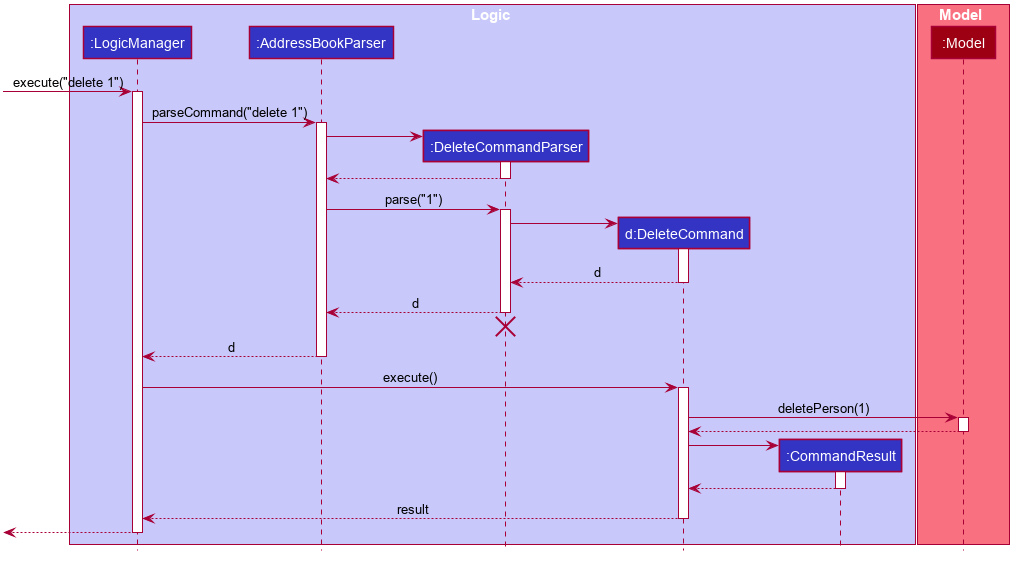
delete 1
Command
The lifeline for DeleteCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
3.7.2. Model component
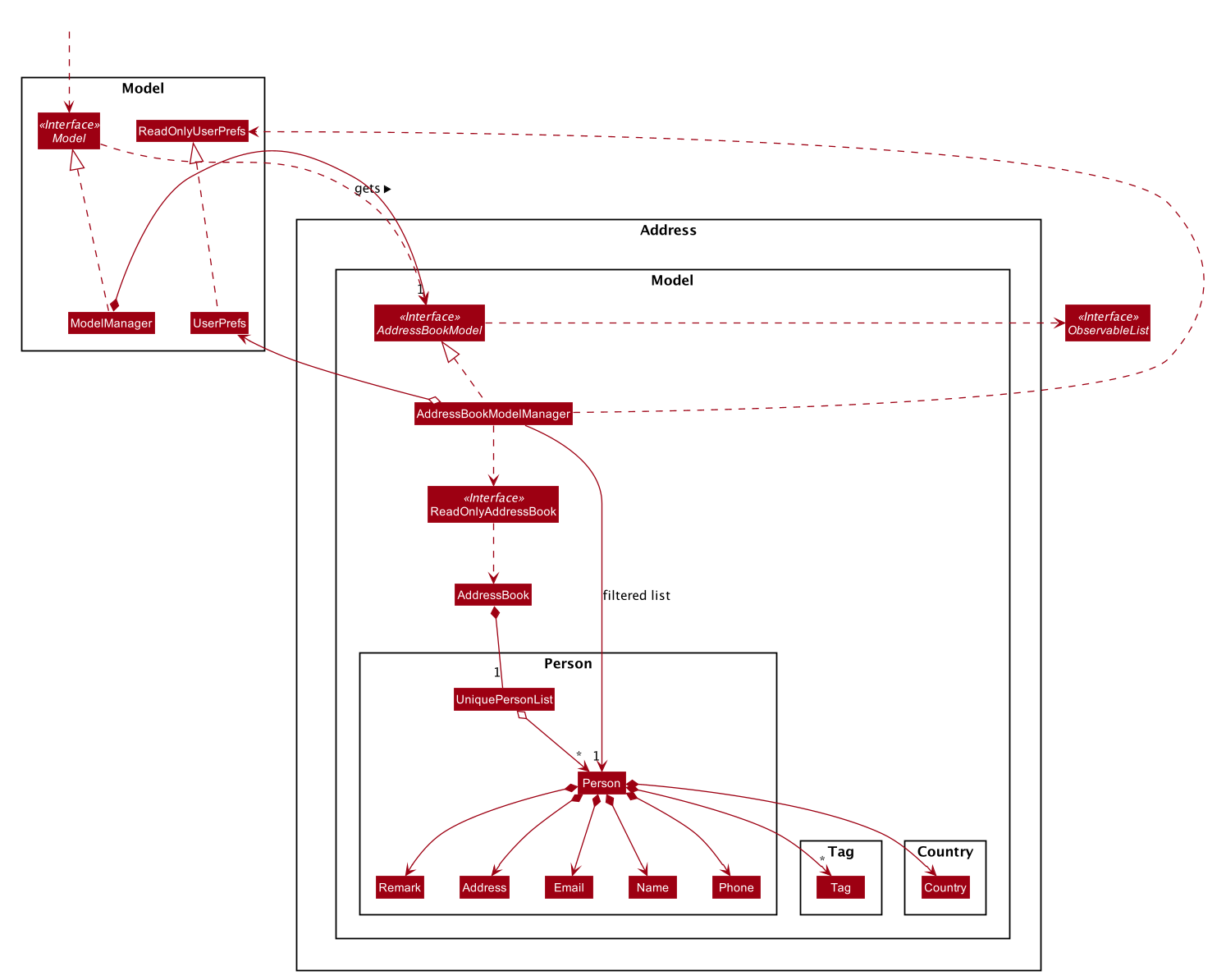
API : AddressBookModel.java
The Model
,
-
stores the
ReadOnlyAddressBook
data andReadOnlyUserPrefs
. -
exposes an unmodifiable
ObservableList<Person>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
As a more OOP model, we can store a Tag list in Address Book , which Person can reference. This would allow Address Book to only require one Tag object per unique Tag , instead of each Person needing their own Tag object. An example of how such a model may look like is given below.![]() |
3.8. Achievements
3.8.1. Logic Component
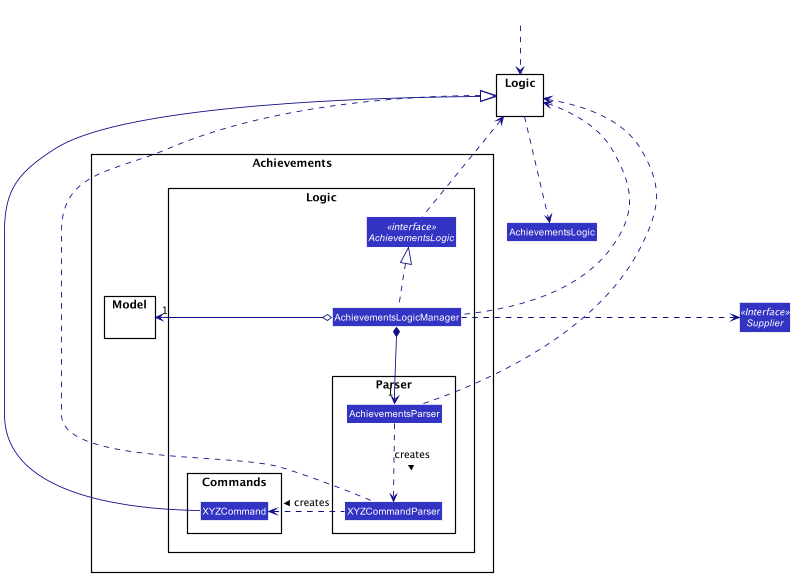
API :
AchievementsLogic.java
The AchievementsLogic
is responsible for generating statistics for the different components in Travezy
-
AchievementsLogicManager
uses theSupplier<StatisticsModel>
to supply theStatisticsModel
. -
Getting the
StatisticsModel
from theSupplier<StatisticsModel>
constructs a newStatisticsModel
that gets the statistics data from a Model from another feature e.g.AddressBookModel
that is visible withinModelManager
-
AchievementsLogicManager
returns the resultant data from theStatisticsModel
that is lazily created.
The AchievementsLogic
parses Command
as well to enable navigation to other Page
within Travezy.
-
AchievementsLogicManager
uses theAchievementsParser
class to parse the user command. -
This results in a
Command
object which is executed by theAchievementsLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the sequence diagram for generating the StatisticsModel
lazily:
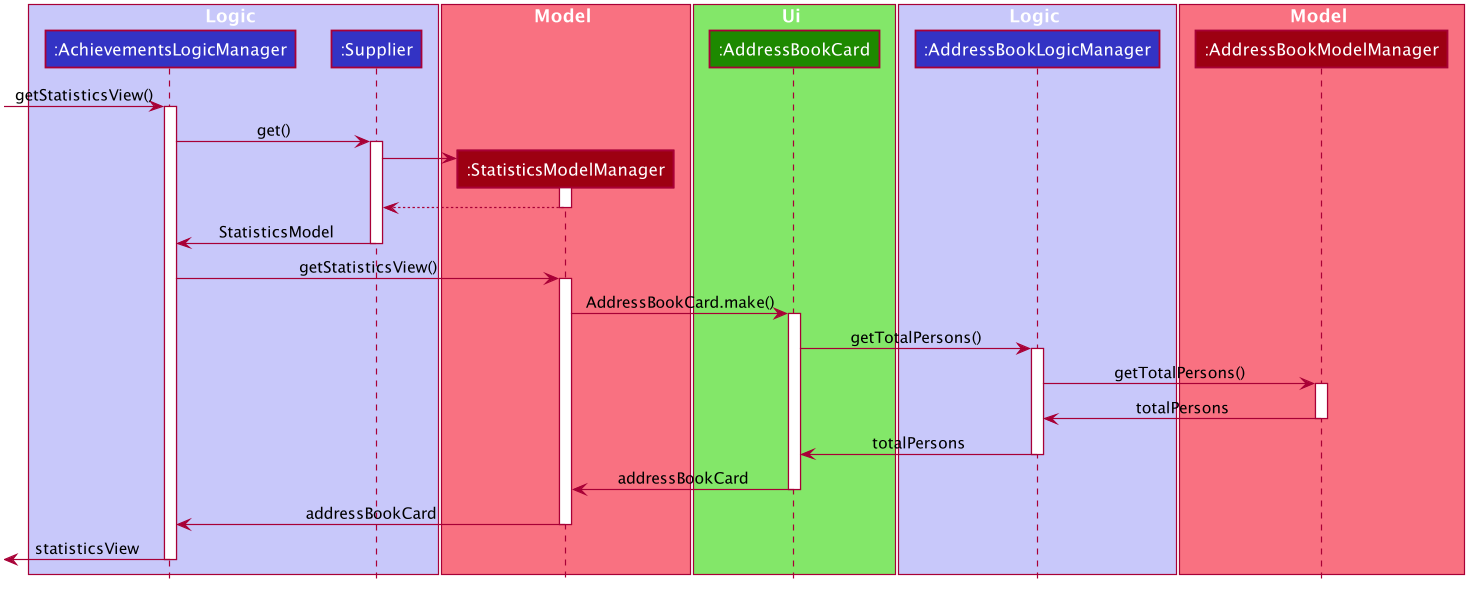
The StatisticsModel is lazily created. Hence, the statistics will only be retrieved from each model only when it is evaluated when loading the AchievementsPage scene.
|
3.8.2. Model Component
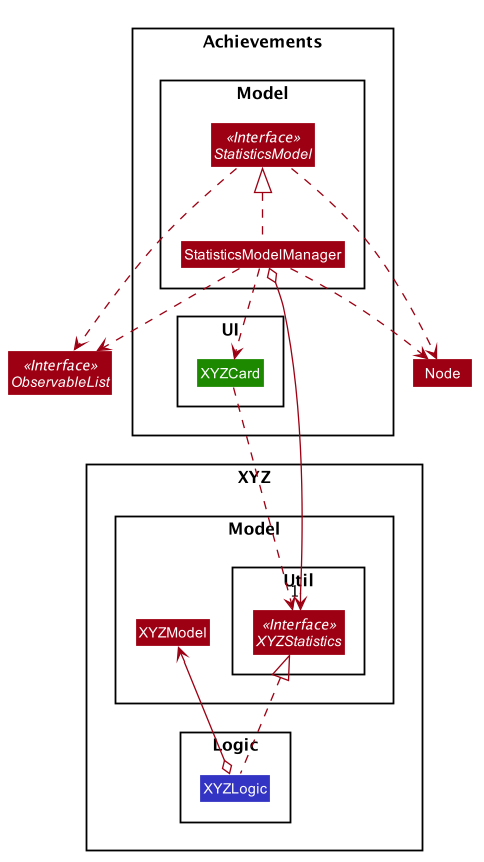
The StatisticsModel
,
-
is constructed from the statistics data from a model of another feature e.g.
AddressBookModel
during instantiation -
contains the statistics data that is generated from a model from another feature e.g.
AddressBookModel
-
does not depend on any of the models from other features / packages e.g.
AddressBookModel
Appendix A: Product Scope
Target user profile:
-
loves to travel frequently
-
travels to many different countries
-
has a need to manage a significant number of contacts from different countries
-
has a need to keep track of travelling expenses
-
lends and pay money to different contacts regularly
-
loves to plan in advance
-
has a need to keep track of different commitments in school
-
love to record his travel itinerary
-
achievements driven
-
has a need to know his/her current progress in completing the achievement
-
motivated to finish his/her accomplishments
-
loves to keep a memory of his/her travels
-
has a need to write down a diary of his/her travel experiences
-
track statistics on inputs (Finances / event and diary entries)
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
-
hates cluttering phone with multiple apps
Value proposition: All-in-one travel manager for planning, scheduling, tracking for a user that loves typing commands.
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
traveller |
record down all my travelling stories. |
my sweet memories stored |
|
student who likes travelling |
record all my spendings on my trip |
keep track of my budgets |
|
NUS student with unusually high workload |
have a calendar planner |
plan ahead |
|
student who needs friends and likes to travel desperately |
manage my travel itineraries |
keep track of my travel schedule during travels |
|
undisciplined individual |
keep track of my expenses |
limit the accumulation of credit card debts |
|
competitive individual |
keep track of my own progress towards different achievements |
challenge myself |
|
student with many friends abroad |
have an Address Book |
contact my friends who are living in that area |
|
student who needs friends and likes to travel desperately |
have an Address Book |
keep my friends’ contact categorized with different countries |
|
NUS student with unusually high workload |
detect conflicts in my calendar schedule |
know when I’m free to travel around the world |
|
undisciplined individual |
keep track of my expenses |
limit the accumulation of credit card debts |
|
user |
hide private contact details by default |
minimize chance of someone else seeing them by accident |
|
user with many persons in the address book |
sort persons by name |
locate a person easily |
|
monolingual individual |
have a language translator |
communicate with others easily |
|
user |
change my application into different themes |
make my application interface look better |
Appendix C: Use Cases
(For all use cases below, the System is TravEzy
and the Actor is the user
, unless specified otherwise)
Use case: Itinerary
Software System : TravEzy Travelling Diary Use Case: UCI01 - Add an event into itinerary page Actor: User Guarantees: . A new event will be added to the itinerary page upon successful command MSS: 1. User requests to list events 2. TravEzy shows a list of events in the itinerary page 3. User type in the event to be added to the itinerary 4. TravEzy add the event in sequential order Extensions 2a. The list is empty. Use case ends. 3a. No event name is given. 3a1. TravEzy shows an error message. Use case resumes at step 2.
Software System : TravEzy Travelling Diary Use Case: UCI02 - Delete an event into itinerary page Actor: User Guarantees: . Specified event will be deleted in the itinerary page upon successful command . Event will not be delete if an invalid index is given MSS: 1. User requests to list events 2. TravEzy shows a list of events in the itinerary page 3. User type in the event to be added to the itinerary 4. TravEzy add the event in sequential order Extensions 2a. The list is empty. Use case ends. 3a. The given index is invalid 3a1. TravEzy shows an error message. Use case resumes at step 2.
Software System : TravEzy Travelling Diary Use Case: UCI03 - Accessing an event Actor: User Precondition: Specified event is contain in the list Guarantees: . Highlights the specific event to be edited MSS: 1. User requests to list events 2. TravEzy shows a list of events in the itinerary page 3. User type in the event to be accessed 4. TravEzy highlights the specified event to be edited Extensions 2a. The list is empty. Use case ends. 3a. The given index is invalid 3a1. TravEzy shows an error message. Use case resumes at step 2.
Software System : TravEzy Travelling Diary Use Case: UCI04 - Add details to event Actor: User Precondition: User must access to an event (UCI03) Guarantees: . Update highlighted event with the details MSS: 1. User requests to edit location / time / description for highlighted event 2. TravEzy prompt for the location / timing / description to be added 3. User inserts the necessary description 4. TravEzy display the changes for that event Extensions 3a. The given location / timing / description is invalid 3a1. TravEzy shows an error message. Use case resumes at step 2. *a. At any time, User chooses to cancel adding details to the event and exit *a1. TravEzy requests to confirm the exit of event *a2. User confirms the exit *a3. TravEzy un-highlights the event selected
Software System : TravEzy Travelling Diary Use Case: UCI05 - Searching for an event Actor: User Precondition: User must add some event into the itinerary (UCI01). So that these events can be searched based on the condition given. Guarantees: . A new event list with the events that met the search conditions. MSS: 1. User requests to search for event. 2. TravEzy display the events which matchese the search description. Extensions 1a. The given search command did not indicate any condition (Title / Date / Time / Location / Description). 1a1. TravEzy shows an error message prompting with the correct format.
Use case: Financial Tracker
Software System : TravEzy Travelling Financial Tracker Use Case: UCFT01 - Add an expense into financial tracker page Actor: User Guarantees: . A new expense will be added to the financial tracker page upon successful command MSS: 1. User type in the expense to be added to the financial tracker 2. TravEzy add the expense in order by date and time Extensions 1a. Command format is incorrect. 1a1. TravEzy shows an error message, prompt user with proper command format. Use case resumes at step 1.
Use case: Calendar
Software System : TravEzy Travelling Calendar Use Case: UCC01 - Add a commitment into the Calendar Actor: User Guarantees: . A new commitment will be added to the calendar page upon successful command MSS: 1. User type in the commitment to be added to the calendar 2. TravEzy add the commitment and visually indicate the commitment on the calendar Extensions 1a. Command format is incorrect. 1a1. TravEzy shows an error message, prompt user with proper command format. Use case resumes at step 1. 1b. The time frame of the commitment clashes with another event that is already in the calendar 1a1. TravEzy will prompt the user whether they would like to proceed with the addition of the event 2a. When the user typed "Yes" Use case resumes at step 2. 2b. When the user typed "No" Use case resumes at step 1.
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
11
or above installed. -
Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
The system should work on both 32-bit and 64-bit environments.
-
The product is not required to produce any file format (Excel, PDF, html) other than JSON file.
Appendix E: Glossary
- Command Line Interface
-
An interface where the user interact with the application through the command box.
- Graphical User Interface
-
A visual display on
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Private contact detail
-
A contact detail that is not meant to be shared with others
- JavaScript Object Notation file
-
More commonly as JSON file is text-based and lightweight which is easily readable and writable.